Line-Line Intersection in AS3
Tuesday, April 28th, 2009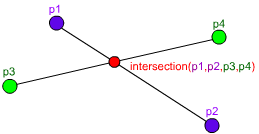
I found a bug in my old Line-Line Intersection in C++ post, and after I fixed it I thought it would be a good idea to port it to AS3:
function intersection(p1:Point, p2:Point, p3:Point, p4:Point):Point { var x1:Number = p1.x, x2:Number = p2.x, x3:Number = p3.x, x4:Number = p4.x; var y1:Number = p1.y, y2:Number = p2.y, y3:Number = p3.y, y4:Number = p4.y; var z1:Number= (x1 -x2), z2:Number = (x3 - x4), z3:Number = (y1 - y2), z4:Number = (y3 - y4); var d:Number = z1 * z4 - z3 * z2; // If d is zero, there is no intersection if (d == 0) return null; // Get the x and y var pre:Number = (x1*y2 - y1*x2), post:Number = (x3*y4 - y3*x4); var x:Number = ( pre * z2 - z1 * post ) / d; var y:Number = ( pre * z4 - z3 * post ) / d; // Check if the x and y coordinates are within both lines if ( x < Math.min(x1, x2) || x > Math.max(x1, x2) || x < Math.min(x3, x4) || x > Math.max(x3, x4) ) return null; if ( y < Math.min(y1, y2) || y > Math.max(y1, y2) || y < Math.min(y3, y4) || y > Math.max(y3, y4) ) return null; // Return the point of intersection return new Point(x, y); }
You can try it here: